Learn how to hide endpoints in API documentation using Swagger in .NET Core, covering both basic and more advanced scenarios. When creating API documentation with Swagger in .NET Core, you may not always want every endpoint to be visible. Certain routes might be reserved for internal or admin use, outdated, or contain sensitive information. By selectively hiding these endpoints, you can simplify your documentation, making it easier for others to understand and navigate.
In this guide, we’ll explore simple and advanced methods to hide Swagger endpoints in .NET Core. We’ll start with a basic option and then dive into custom filtering for more flexibility.
Setting Up Swagger in .NET Core (Using .NET 8)
Before we hide anything, let’s get Swagger running in our project. If you already have Swagger set up, you can skip this section.
To set up Swagger, install the Swashbuckle.AspNetCore NuGet package. This package gives you all the tools you need to create Swagger docs in a .NET Core project. Here’s how to add it:
- Open your terminal or Package Manager Console.
- Run the following command to install Swashbuckle:
dotnet add package Swashbuckle.AspNetCore
Then, go to your Program.cs file and add the Swagger configuration in the Main method:
public static void Main(string[] args)
{
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddControllers();
// Add below metho before app.Run() call
builder.Services.AddSwaggerGen();
var app = builder.Build();
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
// Add below two methods before app.Run() call
app.UseSwagger();
app.UseSwaggerUI();
app.Run();
}
After completing these steps, navigate to /swagger in your browser to view the Swagger UI, which lists all available API endpoints.
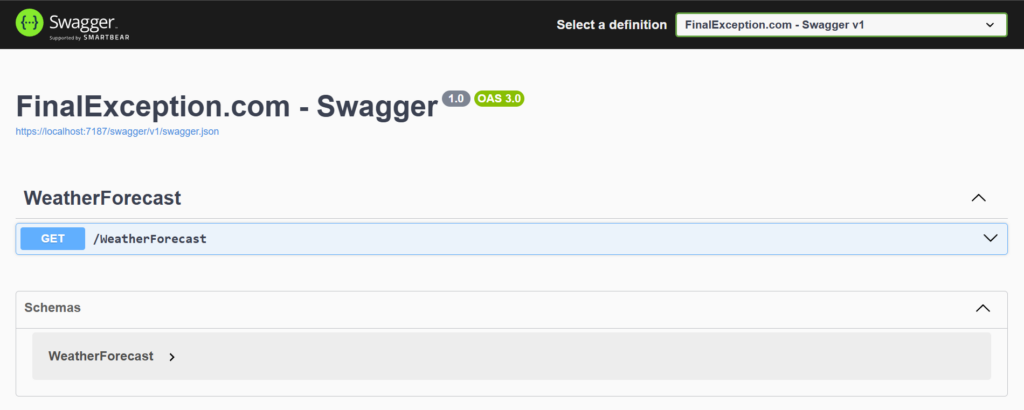
For a more complex setup, check out the example swagger repository at GitHub.
Hiding Endpoints with ApiExplorerSettings
The easiest way to hide specific endpoints in Swagger is by using the ApiExplorerSettings attribute with IgnoreApi = true. This approach is perfect for hiding individual endpoints, such as those used for internal testing.
Here’s an example of how to hide a DELETE endpoint:
[HttpDelete]
[ApiExplorerSettings(IgnoreApi = true)]
public async Task<IActionResult> Delete(int id)
{
await _service.Remove(id);
return NoContent();
}
By adding [ApiExplorerSettings(IgnoreApi = true)], Swagger automatically excludes this endpoint from the documentation. The endpoint remains accessible to the front-end application; it’s simply hidden in Swagger. So, our documentation changed from this
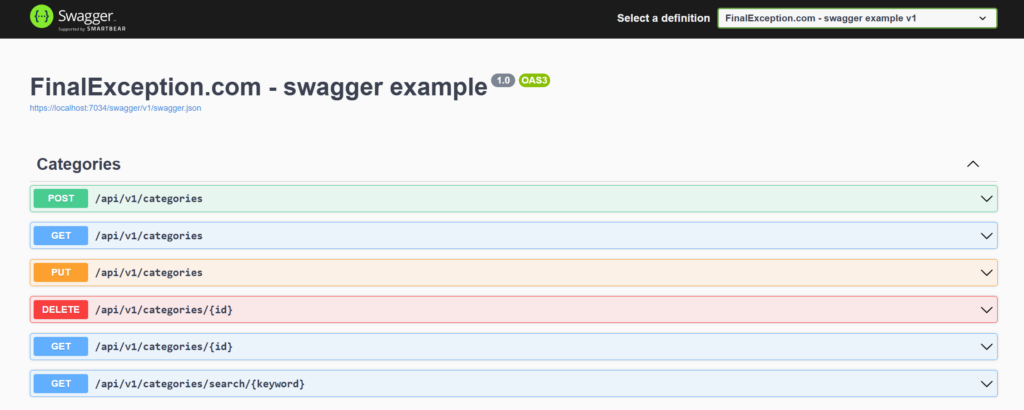
Into this:
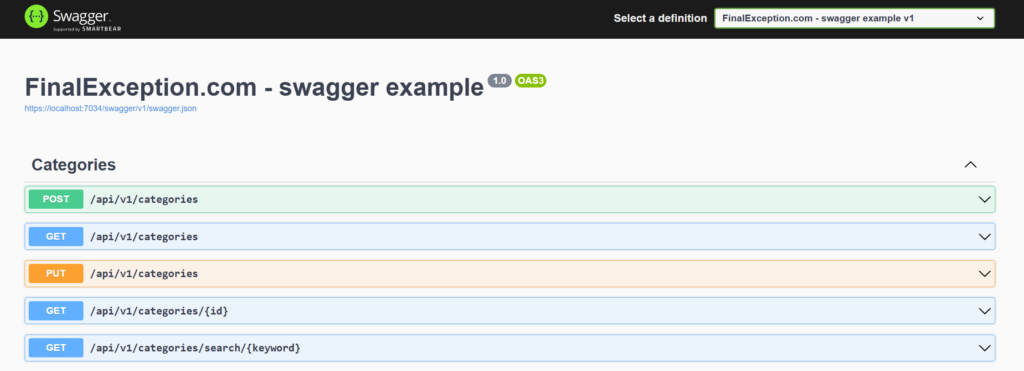
When to Use ApiExplorerSettings attribute
Use ApiExplorerSettings when:
- You only need to hide a few specific endpoints.
- Hidden endpoints are unlikely to change often.
This approach is easy and requires minimal setup, but it’s limited if you need dynamic control over endpoint visibility.
Custom Filtering with a Document Filter
To have more control over which endpoints appear in Swagger, you can create a custom filter. A document filter allows you to write logic that modifies the data that SwaggerUI receives, processes, and displays as a documentation page. Here you can add a filter that will remove a specific endpoint, as many tutorials on the internet recommend, but I think this is a bad solution, since it’s very difficult to remove schemas related to it manually.
To create a custom filter, first you have to create new class, for example, we would like to remove all POST operation. To achiev that by filters, you need to create RemovePostOperationsFilter class, and implement IDocumentFilter:
public class RemovePostOperationsFilter : IDocumentFilter
{
public void Apply(OpenApiDocument swaggerDoc, DocumentFilterContext context)
{
// Go through each path and remove DELETE operations
foreach (var path in swaggerDoc.Paths)
{
path.Value.Operations.Remove(OperationType.Post);
}
}
}
Next, you need to register the filter in Startup.cs
by adding it to SwaggerGen
:
builder.Services.AddSwaggerGen(c =>
{
c.DocumentFilter<RemovePostOperationsFilter>();
});
How DocumentFilter Works
- Implements IDocumentFilter: This enables the Apply method to modify the Swagger document.
- Iterates Through Paths: In the Apply method, swaggerDoc.Paths is looped through. Each path can have multiple HTTP operations (e.g., GET, POST).
- Removes POST Operations: For each path, Operations.Remove(OperationType.Post); removes the POST operation if it exists.
If we run application again and open swagger we will in fact see, that there is no POST request in documentation:
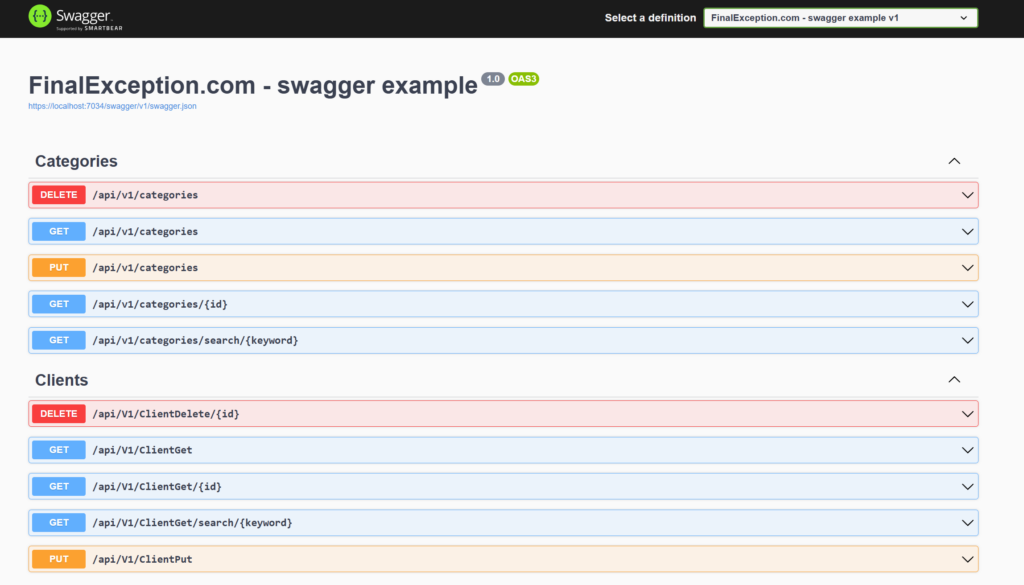
However, if we scoll down the page unti schema section, we will still see the schemas associated to removed endpoints. If we did filtering due to security reasons, we potentialy leak important information about data that we have in our API
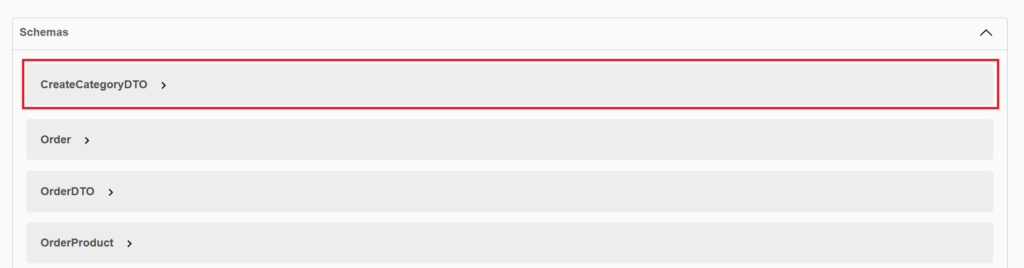
For this very reason, we should avoid using DocumentFilter to filter endpoints. Instead, to remove an operation and its associated data from the documentation, the best option would be to use DocInclusionPredicate
When to use DocumentFilter?
- Grouping Endpoints by Tags: You can dynamically assign tags to endpoints based on specific criteria (e.g., controller names or custom attributes). This helps organize endpoints in Swagger UI for better readability.
- Modifying Descriptions or Metadata: Automatically update descriptions, titles, or other metadata for endpoints based on custom logic, making documentation more consistent without manually updating each endpoint.
Filter endpoints using DocInclusionPredicate
The DocInclusionPredicate
function allows you to control which endpoints appear in the Swagger documentation by including or excluding specific endpoints based on conditions. You typically define it when using Swagger with API versioning or other complex filtering requirements.
In this example, we’ll configure DocInclusionPredicate to exclude any POST endpoints (as in the DocumentFilter) from the Swagger documentation. To do that, we need add a custom condition to exclude all POST endpoints by updating the AddSwaggerGen
configuration.
builder.Services.AddSwaggerGen(c =>
{
// Set up a DocInclusionPredicate to filter out DELETE operations
c.DocInclusionPredicate((docName, apiDesc) =>
{
// Exclude DELETE operations
var httpMethod = apiDesc.HttpMethod?.ToUpper();
return httpMethod != "POST";
});
});
How DocInclusionPredicate works:
- DocInclusionPredicate takes two parameters:
- docName: The document name, often used with API versioning to specify versions.
- apiDesc: The API description, which includes details about the endpoint, such as the HTTP method.
- We check the HTTP method of each endpoint using apiDesc.HttpMethod.
- If the HTTP method is DELETE, we return false, excluding it from Swagger documentation; for all other HTTP methods, we return true to include them.
When we run agin our application and open swagger documentation page, we will see output the same as in document filter section, but this time, in schema section there will be only included schemas that are visible for user, not all of them:
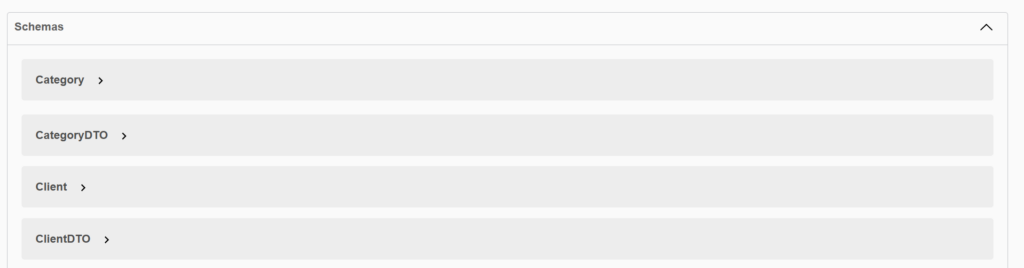
When to use a DocInclusionPredicate?
- You want flexibility over which endpoints appear in Swagger.
- You need to filter out endpoints dynamically based on custom criteria.
- You don’t want to expose sensitive information (like hidden endpoints schemas) in your documentation